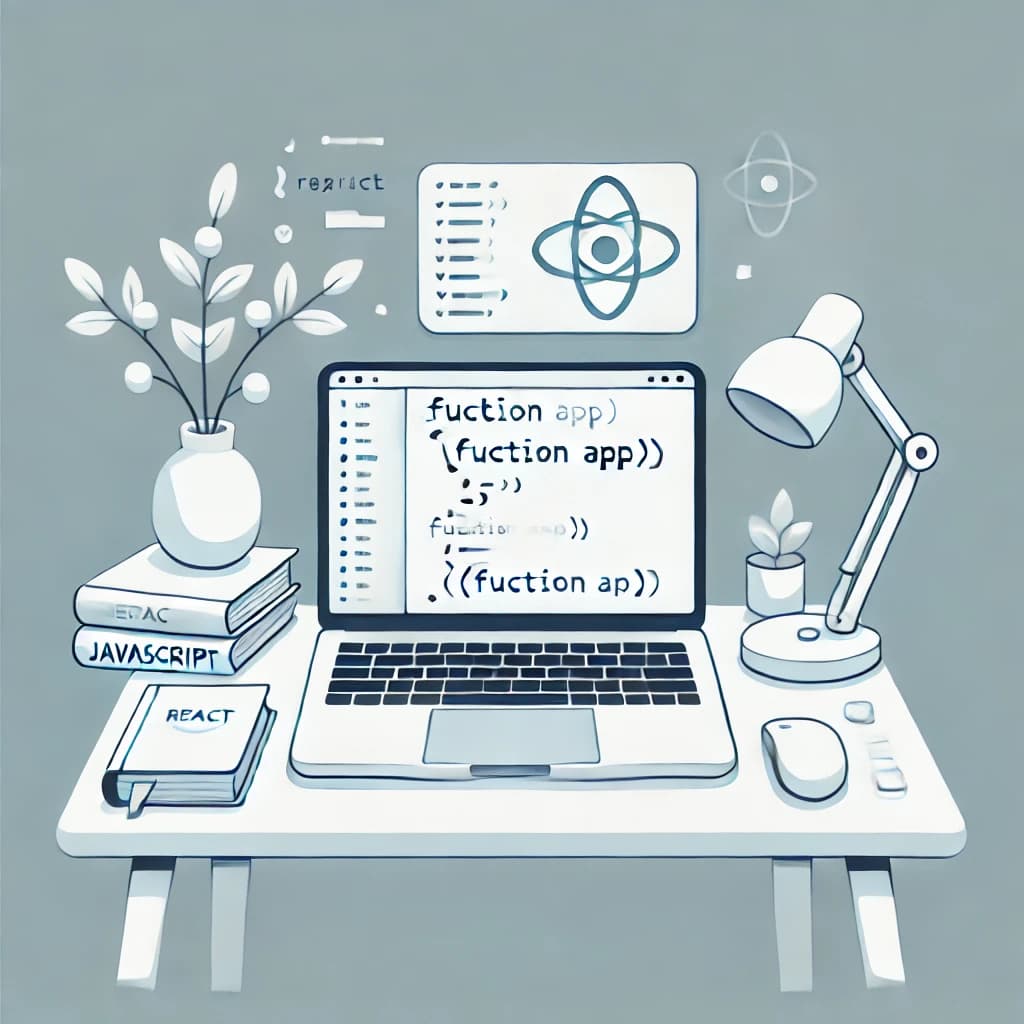
Setting Up React and Useful Icons for Your Project
Bohdan / October 28, 2024
Getting Started with React and Adding Useful Icons
Welcome to this guide on setting up a React application from scratch and enhancing it with popular icon libraries. This tutorial will walk you through everything you need to set up your development environment, create a React project, and seamlessly add icons to enhance the user experience.
Table of Contents
- Why Choose React?
- Setting Up the Development Environment
- Creating a New React App
- Understanding the Project Structure
- Setting Up Components in React
- Using Icons in React
- Installing and Configuring Icon Libraries
- Icon Examples in Action
- Conclusion
Why Choose React?
React is one of the most widely used JavaScript libraries for building user interfaces. Developed by Facebook, React promotes the development of single-page applications (SPAs) that are fast, efficient, and scalable. React’s component-based architecture makes it easy to build complex applications while maintaining modularity.
Setting Up the Development Environment
To start, you’ll need Node.js and npm (Node Package Manager) installed. Verify your installations by running the following commands:
# Verify Node.js installation
node -v
# Verify npm installation
npm -v
If you do not have Node.js and npm, download and install them from the official Node.js website. Creating a New React App
React has a powerful toolchain to help you get started quickly. The Create React App command will scaffold a new project with all the necessary configurations. Run this command to create a new React project:
bash
npx create-react-app my-react-app
This command will create a folder called my-react-app with all the dependencies and configuration files needed. Now, navigate to your project directory:
bash
cd my-react-app
Once inside your project, start the development server:
bash
npm start
Your application will be live at http://localhost:3000, showing a welcome screen. Understanding the Project Structure
Here is an overview of the default project structure:
public/ - Contains static files and the main HTML file. src/ - Houses the JavaScript code, including components, styles, and assets. node_modules/ - Contains all the installed dependencies. package.json - Defines dependencies, scripts, and configurations for the project.
Setting Up Components in React
Components are the building blocks of any React application. Here’s a simple example of a functional component:
jsx
// src/components/Welcome.js
import React from 'react';
function Welcome() {
return <h1>Welcome to React!</h1>;
}
export default Welcome;
Incorporate this component in your App.js file:
jsx
// src/App.js
import React from 'react';
import Welcome from './components/Welcome';
function App() {
return (
<div className="App">
<Welcome />
</div>
);
}
export default App;
This example shows how easy it is to create and use components in React. Using Icons in React
Icons are a critical part of web applications, improving the UI’s usability and design. React allows us to easily incorporate popular icon libraries. Here, we will use React Icons, which provides access to various icon packs, including Font Awesome, Material Design Icons, and Feather Icons. Installing and Configuring Icon Libraries
To install React Icons, use the following command:
bash
npm install react-icons
Now, you can start using icons in your components by importing them from the specific icon library you need. For example, to use an icon from Font Awesome:
jsx
import { FaBeer } from 'react-icons/fa';
function IconExample() {
return (
<div>
<h3>Let’s grab a <FaBeer />!</h3>
</div>
);
}
export default IconExample;
Available Icon Packs
React Icons provides several popular icon packs. Here are a few you might find useful:
Font Awesome (react-icons/fa) Material Design Icons (react-icons/md) Feather Icons (react-icons/fi) Ionicons (react-icons/io)
To view all available icons and packs, visit the React Icons documentation. Icon Examples in Action
Here’s a practical example where we combine a few icons to create an engaging header:
jsx
import { FaHome, FaInfoCircle } from 'react-icons/fa';
import { MdContactMail } from 'react-icons/md';
function Header() {
return (
<header>
<nav>
<a href="/"><FaHome /> Home</a>
<a href="/about"><FaInfoCircle /> About</a>
<a href="/contact"><MdContactMail /> Contact</a>
</nav>
</header>
);
}
export default Header;
In this example, each navigation link has a corresponding icon, making the interface more visually appealing and easier to understand for users. Conclusion
Setting up a React application with React Icons enhances your project with professional-looking icons and ensures a modern user interface. With the flexibility of React Icons, you have access to numerous icon packs that elevate the user experience and make your application stand out.
Happy coding!