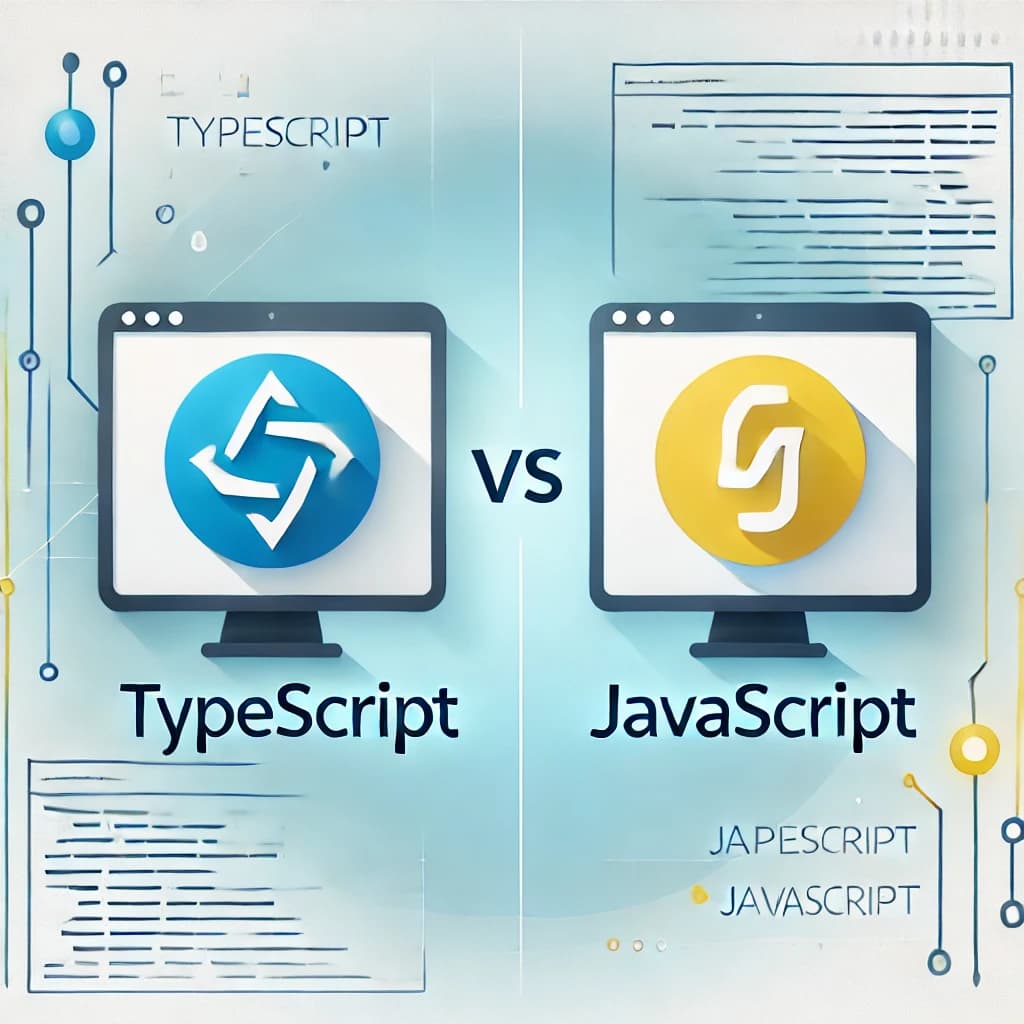
TypeScript vs JavaScript: Advanced Comparison with Tables
Bohdan / October 28, 2024
TypeScript vs JavaScript: Advanced Comparison
Explore the key differences between TypeScript and JavaScript in this guide, complete with a comparison table, interactive code examples, and custom components.
Table of Contents
- Introduction
- JavaScript Overview
- TypeScript Overview
- Feature Comparison Table
- Code Examples
- Interactive Component Example
- Conclusion
Introduction
The choice between JavaScript and TypeScript depends on project requirements, team preferences, and the complexity of the application. This guide provides a detailed comparison of both languages, including interactive elements and tables to illustrate their core differences.
JavaScript Overview
JavaScript is a dynamic, interpreted language widely used for web development. Its flexibility and extensive ecosystem make it the language of choice for building responsive web applications.
JavaScript Features
- Dynamic Typing: Types are assigned at runtime.
- Prototype-based Inheritance: Uses prototypal inheritance rather than classes.
- Asynchronous Programming: Supports promises and async/await.
javascript
// Example JavaScript Code
function greet(name) {
return `Hello, ${name}!`;
}
console.log(greet("Alice")); // Output: Hello, Alice!
TypeScript Overview
TypeScript is a statically typed superset of JavaScript, designed to help developers avoid common errors and enhance code readability. TypeScript’s type-checking, interfaces, and compile-time error reporting make it ideal for larger applications. TypeScript Features
Static Typing: Enforces type definitions at compile time. Enhanced Tooling: Provides autocomplete and error-checking. Support for OOP: Includes classes, interfaces, and enums.
typescript
// Example TypeScript Code
function greet(name: string): string {
return `Hello, ${name}!`;
}
console.log(greet("Alice")); // Output: Hello, Alice!
// console.log(greet(42)); // Type error at compile-time
Feature Comparison Table
Below is a comparison table that highlights the main differences between JavaScript and TypeScript.
Feature | JavaScript | TypeScript |
---|---|---|
Typing | Dynamic (runtime) | Static (compile-time) |
Error Checking | Runtime errors | Compile-time errors |
Tooling | Basic autocomplete | Enhanced autocomplete, refactoring, error-checking |
Compilation | Directly interpreted by browsers | Compiles to JavaScript |
Learning Curve | Lower; easier for beginners | Higher; requires understanding of types and OOP |
Code Examples JavaScript Example
javascript
let message = "Hello";
message = 42; // No error in JavaScript, but this could cause runtime issues
TypeScript Example
typescript
let message: string = "Hello";
// message = 42; // TypeScript will throw a compile-time error
Interactive Component Example
Here’s a live Counter component you can interact with, showcasing MDX interactivity.
Conclusion
Choosing TypeScript or JavaScript depends on your project’s requirements. TypeScript’s type safety and tooling are ideal for large projects, while JavaScript offers flexibility and simplicity for rapid prototyping and smaller applications.
Happy coding!